今回はボリュームを調整を中心に実装して行きます。今回の実装内容は
- スライドバーを用いてボリュームの大きさを調整する。
- Muteボタンを追加して消音機能を追加する。
- 再生中、Play ボタンの色を変える。
前回のスケッチを元に変更して行きます。
#include <Arduino.h>
#include <WiFi.h>
#include "AudioFileSourceICYStream.h"
#include "AudioFileSourceBuffer.h"
#include "AudioGeneratorMP3.h"
#include "AudioOutputI2S.h"
#include <WebServer.h>
#include <ESPmDNS.h>
// Enter your WiFi setup here:
const char *SSID = "XXXXXX";
const char *PASSWORD = "YYYYYY";
// Randomly picked URL
const char *URL="http://199.180.75.118:80/stream";
AudioGeneratorMP3 *mp3;
AudioFileSourceICYStream *file;
AudioFileSourceBuffer *buff;
AudioOutputI2S *out;
int flg=0;
int vol=50;
WebServer server(80);
String index_Dt =
"<!DOCTYPE html>\n<html>\n<head>\n"
"<title>ESP32 WebRadio</title>\n</head>\n"
"<body>\n<center>\n<h2>ESP32 WebRadio</h2>\n"
"<form id='cmd_vol' method='get'>\n"
"<input type='range' min='0' max='100' step='1' name='3' onchange='showValue()' id='s_value' value='50'>\n"
"<span id='p_value'>50</span><br><br>\n"
"</form>\n"
"<form method='get'>\n"
"<button type='submit' name='1' id='b_play' style='background:#90ee90'>PLAY</button>\n"
"<button type='submit' name='2' style='background:#90ee90'>STOP</button>\n"
"<button type='submit' name='4' id='b_mute' style='background:#90ee90'>MUTE</button>\n"
"</form>\n"
"<script>\n"
"function showValue () {\n"
"target = document.getElementById('cmd_vol');\n"
"target.submit();\n}\n";
void setup()
{
Serial.begin(115200);
delay(1000);
Serial.println("Connecting to WiFi");
WiFi.disconnect();
WiFi.softAPdisconnect(true);
WiFi.mode(WIFI_STA);
WiFi.begin(SSID, PASSWORD);
// Try forever
while (WiFi.status() != WL_CONNECTED) {
Serial.println("...Connecting to WiFi");
delay(1000);
}
Serial.println("Connected");
Serial.println(SSID);
Serial.print("IP address: ");
Serial.println(WiFi.localIP());
if (MDNS.begin("esp32")) {
Serial.println("MDNS responder started");
}
server.on("/", handleRoot);
server.begin();
Serial.println("HTTP server started");
out = new AudioOutputI2S();
}
void loop()
{
if(flg)
{
if (mp3->isRunning()) {
if (!mp3->loop()) mp3->stop();
}
else {
Serial.printf("MP3 done\n");
delay(1000);
}
}
server.handleClient();
}
void handleRoot()
{
String cmd;
int a;
cmd=server.argName(0);
switch(cmd.toInt())
{
case 1: // Play
if(flg == 0)
{
file = new AudioFileSourceICYStream(URL);
buff = new AudioFileSourceBuffer(file, 2048*16);
mp3 = new AudioGeneratorMP3();
mp3->begin(buff, out);
flg=1;
}
break;
case 2: // Stop
if (mp3) { mp3->stop(); delete mp3; mp3 = NULL; }
if (buff) { buff->close(); delete buff; buff = NULL; }
if (file) { file->close(); delete file; file = NULL; }
flg=0;
break;
case 3: // Volume
cmd=server.arg("3");
vol=cmd.toInt();
out->SetGain((float)vol/100.0);
break;
case 4: // Mute
vol *= -1;
a=vol;
if(vol < 0) a=0;
out->SetGain(((float)a)/100.0);
break;
}
cmd=index_Dt;
cmd += ("document.getElementById('s_value').value='" + String(abs(vol)) + "'\n");
cmd += ("document.getElementById('p_value').innerHTML='" + String(abs(vol)) + "'\n");
if(flg)
cmd += ("document.getElementById('b_play').style.backgroundColor='#ff6347'\n");
if(vol < 0)
cmd += ("document.getElementById('b_mute').style.backgroundColor='#2020ff'\n");
cmd += "</script>\n</center>\n</body>\n</html>";
server.send(200, "text/html",cmd);
}
- 24行:int vol=50; ボリューム用変数
- 33から41行:0から100までのスライドバーを設定。
- PLAY STOP MUTE ボタンの設定。
- 44から47行:スライドバーが変更になった時にサーバーに値を送る Script
- 126から130行:ボリュームを設定するルーチン。スライドバーが変更されサーバに値が送られとこのルーチンに来る。
- 127行:cmd=server.arg(“3”); クライアントから3=XXXとデータが送られてくるので、XXXをcmdに代入。
- 128行:vol=cmd.toInt(); 文字、”XXX” を数字に変換してvolに代入。
- 129行:out->SetGain((float)vol/100.0); これが音量を設定する関数。volの値0から100で音量を調整する。
- 132から137行: MUTE(消音)処理
- 133行:vol *= -1; Muteボタンが押されたらMuteの符号を反転させる。値が負ならMUTE中と判断。
- 136行:out->SetGain(((float)a)/100.0); Mute中ならa=0となり音が出ない。
- 141から147行: スライダーの位置、値。PLAY、MUTEボタンの色設定
- 141行:スライダの位置を設定
- 142行:スライダの値を表示
- 143,144行:PLAYボタンの色設定。再生中ー>オレンジ。停止ー>水色
- 145,146行:MUTEボタンの色設定。OFFー>水色。 ONー>青
コンパイル、実行、ブラウザに、”esp32.local”と入力して下さい。この画面が出ると思います。
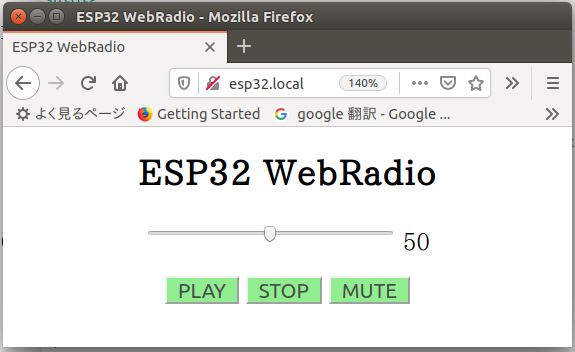
このページのソースは
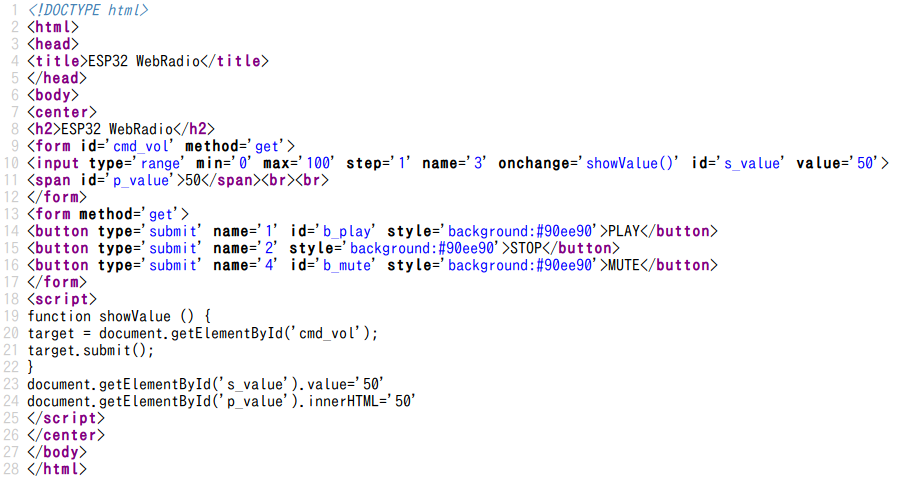
ちなみにスライドバーを移動すると、
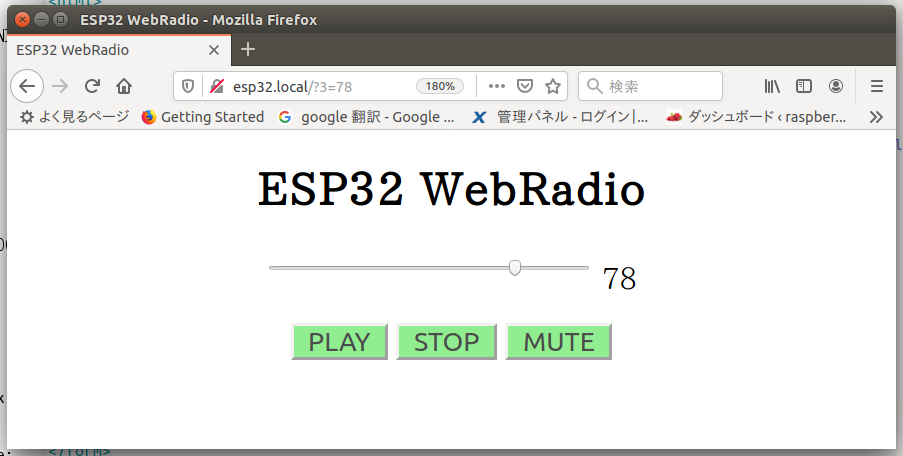
23,24行のコードが変わります。
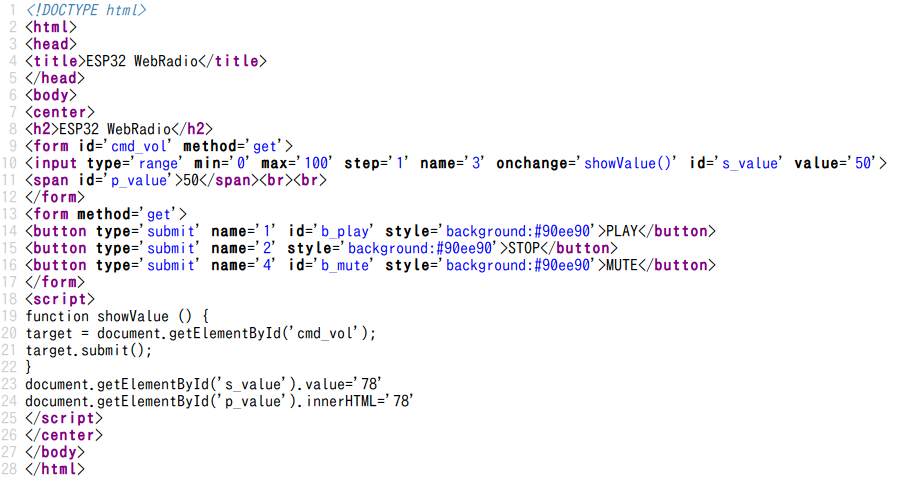
サーバ側でスケッチの141,142行でバーの値に合わせてHTMLののコード書き換えています。
PLAYボタンやMUTEボタンの色も同じ要領で変えています。ボタンの色に合わせてHTMLが増えたり減ったりしています。PLAYボタンを押した場合
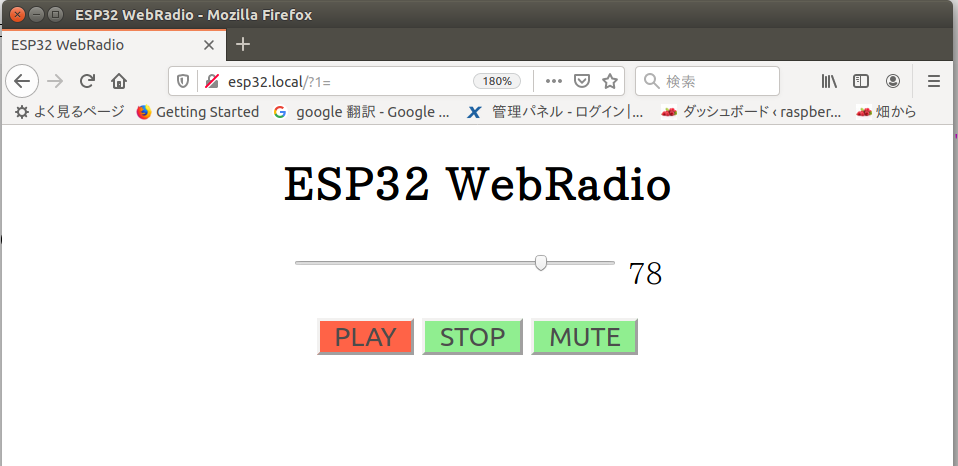
PLAYボタンがオレンジに変わり、ソースは
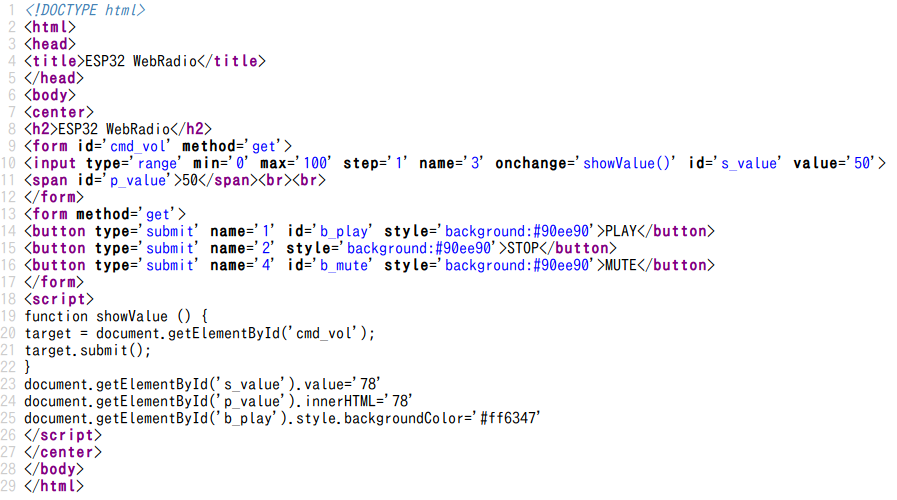
25行目にボタンの色を変更するHTMLが足されています。
これで音量調整の実装は終わり。次回は選局の実装を行いたいと思います。